functions.phpの中でよく使うコード
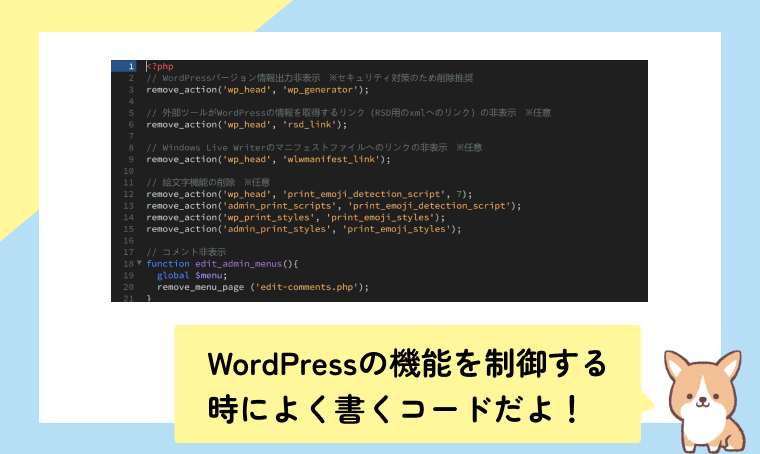
wp_headで出力された不要なコードを省く
// WordPressバージョン情報出力非表示 ※セキュリティ対策のため削除推奨
remove_action('wp_head', 'wp_generator');
// 外部ツールがWordPressの情報を取得するリンク(RSD用のxmlへのリンク)の非表示 ※任意
remove_action('wp_head', 'rsd_link');
// Windows Live Writerのマニフェストファイルへのリンクの非表示 ※任意
remove_action('wp_head', 'wlwmanifest_link');
// 絵文字機能の削除 ※任意
remove_action('wp_head', 'print_emoji_detection_script', 7);
remove_action('admin_print_scripts', 'print_emoji_detection_script');
remove_action('wp_print_styles', 'print_emoji_styles');
remove_action('admin_print_styles', 'print_emoji_styles');
管理画面ログインページのロゴを変更
function my_login_logo(){
echo
'<style>
body{background: #f8f8f8!important;} /* ログイン画面背景色を指定 */
body.login div#login h1 a {
background: url('. get_stylesheet_directory_uri() .'/img/site-id-img.svg) no-repeat center; /* 画像を指定 */
background-size: contain;
width: 100px; /* 横幅を指定 */
height: 100px; /* 縦幅を指定 */
margin-bottom: 0;
}
</style>';
}
add_action('login_enqueue_scripts', 'my_login_logo');
アイキャッチ画像の設定領域を追加
function theme_setup(){
add_theme_support('post-thumbnails');
add_image_size('article-thumbnail', 250, 250, true); // ※任意 自分が指定したサイズに切り出したい場合に名前、サイズを指定
}
add_action('after_setup_theme', 'theme_setup');
CSSの読み込み
※<?php wp_head(); ?>を記載している場所に出力
// CSS読み込み
function my_stylesheet(){
// 共通のreset.cssを読み込む
wp_enqueue_style('reset', get_stylesheet_directory_uri() .'/css/reset.css', array(), '1.0.0', false); // faleseの場合、<head>の中に表示
// style.cssを読み込む、array('reset')と指定してreset.cssより下に表示させる
wp_enqueue_style('main-style', get_stylesheet_directory_uri() .'/style.css', array('reset'), '1.0.0', false);
// 各ページで使用するCSSを条件分岐 ※不要なら削除
if(is_page('company')):
wp_enqueue_style('company', get_stylesheet_directory_uri() .'/css/company.css', array(), '', false);
elseif(is_single() || is_category()):
wp_enqueue_style('single', get_stylesheet_directory_uri() .'/css/single.css', array(), '', false);
endif;
}
add_action('wp_enqueue_scripts', 'my_stylesheet');
JavaScriptの読み込み
※<?php wp_head(); ?>を記載済みの状態で、<?php wp_footer(); ?>を記載している場所に出力
// JavaScript読み込み
function my_scripts(){
wp_enqueue_script('jquery-min-js', 'https://code.jquery.com/jquery-3.6.4.min.js', array(), '3.6.4', true); // trueの場合、</body>の前に表示
wp_enqueue_script('main-script', get_stylesheet_directory_uri() .'/js/script.js', array('jquery-min-js'), '1.0.0', true);
// 各ページで使用するJavaScriptを条件分岐 ※不要なら削除
if(is_page('company')):
wp_enqueue_script('company', get_stylesheet_directory_uri() .'/js/company.js', array('jquery-min-js'), '', true);
elseif(is_single() || is_category()):
wp_enqueue_script('single', get_stylesheet_directory_uri() .'/js/single.js', array('jquery-min-js'), '', true);
endif;
}
add_action('wp_enqueue_scripts', 'my_scripts');
WordPressに登録されているjQuery読み込む
※WordPressに登録されているjQueryを読み込む場合、自作のjsに「$」を使用していれば「jQuery」に変更する必要があります。
例:$(‘#gnav’).toggleClass(‘is-open’); → jQuery(‘#gnav’).toggleClass(‘is-open’);
function my_scripts_method(){
wp_enqueue_script('jquery');
}
add_action('wp_enqueue_scripts', 'my_scripts_method');
WordPressに登録されている jQuery を出力させない
function my_scripts_method(){
wp_deregister_script('jquery');
}
add_action('wp_enqueue_scripts', 'my_scripts_method');
管理画面で投稿の「タグ」をチェックボックスで選択できるように見た目を変更
function change_post_tag_to_checkbox(){
$args = get_taxonomy('post_tag');
$args -> hierarchical = true; // Gutenberg用
$args -> meta_box_cb = 'post_categories_meta_box'; // Classicエディター用
register_taxonomy('post_tag', 'post', $args);
}
add_action('init', 'change_post_tag_to_checkbox', 1);
管理画面のメニューからコメントを非表示
//コメント非表示
function edit_admin_menus() {
global $menu;
remove_menu_page ( 'edit-comments.php' );
}
add_action( 'admin_menu', 'edit_admin_menus' );
管理画面の「投稿」の名前を変更
// 投稿の名前変更
function change_menu_label(){
global $menu;
global $submenu;
$name = 'お知らせ';
$menu[5][0] = $name;
$submenu['edit.php'][5][0] = $name.'一覧';
$submenu['edit.php'][10][0] = '新しい'.$name;
}
function change_object_label(){
global $wp_post_types;
$name = 'お知らせ';
$labels = &$wp_post_types['post']->labels;
$labels->name = $name;
$labels->singular_name = $name;
$labels->add_new = _x('追加', $name);
$labels->add_new_item = $name.'の新規追加';
$labels->edit_item = $name.'の編集';
$labels->new_item = '新規'.$name;
$labels->view_item = $name.'を表示';
$labels->search_items = $name.'を検索';
$labels->not_found = $name.'が見つかりませんでした';
$labels->not_found_in_trash = 'ゴミ箱に'.$name.'は見つかりませんでした';
}
add_action('init', 'change_object_label');
add_action('admin_menu', 'change_menu_label');
管理画面のメニューの並び替え
function original_menu_order($menu_order){
if(!$menu_order) return true;
return array(
'index.php', // ダッシュボード
'separator1', // 区切り線
'edit.php', // 投稿
'edit.php?post_type=en-news', // カスタム投稿タイプ en-news
'edit.php?post_type=works', // カスタム投稿タイプ works
'edit.php?post_type=page', // 固定ページ
'upload.php', // メディア
'themes.php', // 外観
'plugins.php', // プラグイン
'users.php', // ユーザー
'tools.php', // ツール
'options-general.php', // 設定
);
}
add_filter('custom_menu_order', 'original_menu_order');
add_filter('menu_order', 'original_menu_order');
カスタム投稿タイプの追加
function create_post_type(){
// カスタム投稿タイプ名 diary 追加
register_post_type(
'diary', // カスタム投稿タイプ名
array(
'labels' => array( // 管理画面上の表示
'name' => '日記',
'singular_name' => '日記',
),
'public' => true, // 管理画面に表示するかどうかの指定
'menu_position' => 5, // メニュー位置の指定
'has_archive' => true, // 投稿した記事のアーカイブページを生成
'show_in_rest' => true, // Gutenbergエディターの有効化
'supports' => array('title', 'editor', 'thumbnail', 'revisions', 'excerpt') // 編集画面に表示させる項目の指定
)
);
// カスタム投稿タイプ名 diary のカスタムタクソノミー追加
register_taxonomy(
'diary-cat', // カスタムタクソノミー diary-catの名前
'diary', // カスタムタクソノミーを追加したいカスタム投稿タイプ名 diaryを記載
array(
'label' => 'カテゴリー', // 管理画面表示名称
'public' => true, // 管理画面に表示するかどうかの指定
'hierarchical' => true, // 階層を持たせるかどうか ※タグの追加の場合は false
'show_in_rest' => true, // REST APIの有効化。ブロックエディタの有効化。
)
);
// flush_rewrite_rules( false ); ※カスタム投稿タイプの記事が404エラーになった場合はこのコードを記述すると直ることがあります
}
add_action( 'init', 'create_post_type' );
カスタムメニューを追加
※出力の仕方はheader.phpとfooter.phpを参照
// カスタムメニューを追加
function register_my_menus(){
register_nav_menus(array( // 複数のナビゲーションメニューを登録する時の関数
// 「メニューの位置」の識別子 => メニューの説明の文字列,
'main-menu' => 'Main Menu', // グローバルナビゲーション用
'footer-menu' => 'Footer Menu', // フッターナビゲーション用
));
}
add_action('after_setup_theme', 'register_my_menus');
ウィジェット機能を追加
※出力の仕方はsidebar.phpとfooter.phpを参照
// ウィジェット機能を追加
function my_widgets_init(){
register_sidebar(array(
'name' => 'News Widgets', // News用のウィジェット名
'id' => 'news-widgets', // News用のウィジェットID名
'before_widget' => '<div id="%1$s" class="widget">', // ※必要なら追加 <ul>で出力されるソースコードを<div>に変更
'after_widget' => '</div>', // ※必要なら追加 </ul>で出力されるソースコードを</div>に変更
));
register_sidebar(array(
'name' => 'Footer Widgets', // Footer用のウィジェット
'id' => 'footer-widgets', // Footer用のウィジェットID名
'before_widget' => '<div id="%1$s" class="widget">', // ※必要なら追加 <ul>で出力されるソースコードを<div>に変更
'after_widget' => '</div>', // ※必要なら追加 </ul>で出力されるソースコードを</div>に変更
));
}
add_action('widgets_init', 'my_widgets_init');
保護中のページのタイトルから「保護中:」を削除し、テキストを変更
//保護中のページのタイトルから「保護中:」を削除
add_filter('protected_title_format', 'remove_protected');
function remove_protected($title) {
return '%s';
}
//保護中のテキストを変更
function my_password_form() {
return
'<p>パスワードを入力してください。</p>
<form class="post-password" action="' . home_url() . '/wp-login.php?action=postpass" method="post"><input name="post_password" type="password" size="24" /><input type="submit" name="Submit" value="' . esc_attr__("パスワードを送信") . '" /></form>';
}
add_filter('the_password_form', 'my_password_form');
最低限覚えておきたい
WordPressのコード
-
PHPの条件分岐の基本
-
WordPressで使う代表的な条件分岐
-
投稿の出力でよく使うWordPressのコード
-
テンプレートパーツの読み込みで使うWordPressのコード
-
header.phpの中でよく使うWordPressのコード
-
footer.phpの中でよく使うWordPressのコード
-
sidebar.phpの中でよく使うWordPressのコード
-
front-page.phpでよく使うWordPressのコード
-
page.phpの中でよく使うWordPressのコード
-
archive.php(date.php、category.php、tag.php)の中でよく使うWordPressのコード
-
single.phpの中でよく使うWordPressのコード
-
archive-カスタム投稿名.phpの中でよく使うWordPressのコード
-
taxonomy.phpの中でよく使うWordPressのコード
-
single-カスタム投稿名.phpの中でよく使うWordPressのコード
-
search.phpの中でよく使うWordPressのコード
-
プラグイン出力でよく使うコード